Identifiers and Variables
Identifiers are the names that identify the elements such as classes, methods, and variables in a program.
All identifiers must obey the following rules:
An identifier is a sequence of characters that consists of letters, digits, underscores(_), and dollar signs ($).
An identifier must start with a letter, an underscore (_), or a dollar sign ($). It cannotstart with a digit.
An identifier cannot be a reserved word. (See Appendix A for a list of reserved words.)
An identifier cannot be true, false, or null.
An identifier can be of any length.
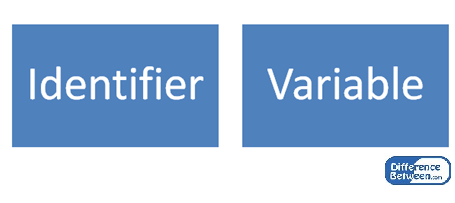
All identifiers must obey the following rules:
For example, $2, ComputeArea, area, radius, and print are legal identifiers, whereas
2A and d+4 are not because they do not follow the rules.
Since Java is case sensitive, area, Area, and AREA are all different identifiers.
Variables are used to store values that may be changed in the program.
The variable declaration tells the compiler to allocate appropriate memory space for the variable based on its data type. A variable must be declared before it can be assigned a value.
The syntax for declaring a variable is
datatype variableName;
Here are some examples of variable declarations:
int count; // Declare count to be an integer variable
double radius; // Declare radius to be a double variable
double interestRate; // Declare interestRate to be a double variable
You can also use a shorthand form to declare and initialize variables of the same type together. For example,
int i = 1, j = 2;
For example, radius and area are variables of the double type. You can assign any numerical value to radius
and area in the following code, radius is initially 1.0 (line 2) and then changed to 2.0 (line 7), and area is set to
3.14159 (line 3) and then reset to 12.56636 (line 8).
Thanks And Regards
Dhirenkumar Rathod....
Comments
Post a Comment